39 how to update a label in tkinter
How do I create an automatically updating GUI using Tkinter in Python? from Tkinter import * from random import randint root = Tk() lab = Label(root) lab.pack() def update(): lab['text'] = randint(0,1000) root.after(1000, update) # run itself again after 1000 ms # run first time update() root.mainloop() This will automatically change the text of the label to some new number after 1000 milliseconds. tkinter - How to update a label in python? - Stack Overflow the click=StringVar (), because when a set () command on this object is invoked, it counts like an event, and so the label including it is updated. - LMD Jan 14, 2017 at 14:04 Show 1 more comment
Tkinter how to continuously update a label - Stack Overflow root.after (1000, Update) Secondly, you need some way to make the update happen every second, rather than just once. The easiest way is to add a root.after call to your Update function, as well as calculating the new time string each time the function is called:
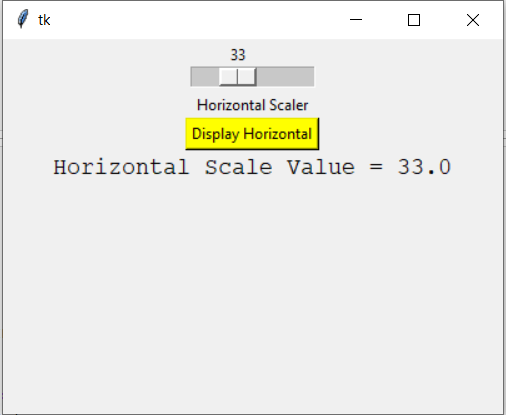
How to update a label in tkinter
How to update the image of a Tkinter Label widget? - tutorialspoint.com In the following example, we will create a button to update the Label image. #Import the required library from tkinter import* from PIL import Image, ImageTk #Create an instance of tkinter frame win= Tk() #Define geometry of the window win.geometry("750x600") win.title("Gallery") #Define a Function to change to Image def change_img(): How to update a Python/tkinter label widget? - tutorialspoint.com Output. Running the above code will display a window that contains a label with an image. The Label image will get updated when we click on the "update" button. Now, click the "Update" button to update the label widget and its object. How to update Tkinter labels using a button. - Python We don't have the file so don't know what it contains, nor did you say how you want the labels updated. Initially you open "stats" as read only and never close it. When you open it again in the write() function, the result is unpredictable because the file is already open.
How to update a label in tkinter. Tkinter Label - Python Tutorial First, import Label class from the tkinter.ttk module. Second, create the root window and set its properties including size, resizeable, and title. Third, create a new instance of the Label widget, set its container to the root window, and assign a literal string to its text property. Setting a specific font for the Label Change the Tkinter Label Text | Delft Stack Use StringVar to Change/Update the Tkinter Label Text StringVar is one type of Tkinter constructor to create the Tkinter string variable. After we associate the StringVar variable to the Tkinter widgets, Tkinter will update this particular widget when the variable is modified. Python Tkinter - Label - GeeksforGeeks textvariable: As the name suggests it is associated with a Tkinter variable (usually a StringVar) with the label. If the variable is changed, the label text is updated. bitmap:It is used to set the bitmap to the graphical object specified so that, the label can represent the graphics instead of text. fg:The label clior, used for text and bitmap ... python - Tkinter Label refresh problem [SOLVED] | DaniWeb You can manually update a label also. This example is from somewhere on the web and should use a class like above, but should show you the technique to be used. from Tkinter import * root=Tk() def changeLabel(): myString.set("I'm, a-fraid we're fresh out of red Leicester, sir. ") myString=StringVar() Label(root,textvariable=myString).pack ...
python - Tkinter, cant update label in realtime - Stack Overflow Is it possible to update the label in realtime instead of closing and opening the new window to see a change in the counter? Thankful for all help I can get. Please see code below: from tkinter import *. root = Tk () root.geometry ("300x300") count = 20. def ACdriver (option): How to update a tkinter Label()? - Treehouse How to update a tkinter Label()? I'm working on a pretty complicated program for a prototype of an app. But I am not so great with tkinter, and because I was having a couple of problems with updating Label() objects, I made a little program to try that. My code for that little program is: Update a Label while the app is running without a button on Tkinter ... 1 from tkinter import * 2 from tkinter import scrolledtext 3 4 root = Tk() 5 6 dataFrame = Frame(root) 7 recFrame = Frame(root) 8 9 dataLabel = Label(root, text="Dados").grid(column=0, row=0) 10 dataInput = scrolledtext.ScrolledText(root, width=3, height=10) 11 dataInput.grid(column=0, row=1) 12 13 dataFrame.grid(column=0) 14 15 How to Change Label Text on Button Click in Tkinter Change Label Text Using 'text' Property Another way to change the text of the Tkinter label is to change the 'text' property of the label. import tkinter as tk def changeText(): label['text'] = "Welcome to StackHowTo!" gui = tk.Tk() gui.geometry('300x100') label = tk.Label(gui, text="Hello World!") label.pack(pady=20)
Tkinter update label with variable from inside function - CMSDK Tkinter Variables are different from normal variables. To create one: label_text = tk.StringVar() Then, rather than assigning to the variable, you nee to use the set method:. label_text.set('') or. label_text.set('Not Valid') python - [tkinter] update label every n seconds | DaniWeb There is more than one way to skin that cat. I use a tkinter variable and update it. Note that you have to call updater () to get the whole thing started. try: import Tkinter as tk ## Python 2.x except ImportError: import tkinter as tk ## Python 3.x class UpdateLabel(): def __init__(self): self.win = tk.Tk() self.win.title("Ausgangsposition ... Updating a label in Python tkinter! Please help :-) - CodeProject Solution 3. It is because tkinter window closed but other processes related to it e.g. Python. Copy Code. answerLabel.destroy () is still running. To avoid this, put try and except when calling answer () function. To avoid the error, do this whenever answer () is called: Python. Tkinter Change Label Text - Linux Hint from tkinter import * window1 = Tk () text1 = "Tkinter Change Label Text Example" def counter (): global text1 label1. config( text = text1) button1 = Button ( window1, text = "Update Text", command = counter) label1 = Label ( window1, text = "Tkinter Change Label Text") label1. pack() button1. pack() window1. mainloop()
How to dynamically add/remove/update labels in a Tkinter window? To dynamically update the Label widget, we can use either config (**options) or an inline configuration method such as for updating the text, we can use Label ["text"]=text; for removing the label widget, we can use pack_forget () method. Example
Tkinter: How to update widgets using value selected in Combobox ... To get value selected in Combobox and use it to update value in Label you can use bind () with (virtual) event '<>'. It can be used to assing function which will be executed you select value in Combobox - and this function may update value in Label. Next you use bind () to assign function's name - ie. on_select - (without ...
Updating tkinter labels in python - TechTalk7 Updating tkinter labels in python By user user August 29, 2021 In python, tkinter 2 Comments I'm working on giving a python server a GUI with tkinter by passing the Server's root instance to the Tkinter window. The problem is in keeping information in the labels up to date.
How to make Python tkinter label widget update? - The Web Dev To make Python tkinter label widget update, we assign the textvariable argument a StringVar object before we call set to update the label. v = StringVar () Label (master, textvariable=v).pack () v.set ("New Text!") We set textvariable to a StringVar object to make set update the label. Then we call set to update the label to display 'New Text
How to change the Tkinter label text? - GeeksforGeeks Click here For knowing more about the Tkinter label widget. Now, let' see how To change the text of the label: Method 1: Using Label.config () method. Syntax: Label.config (text) Parameter: text - The text to display in the label. This method is used for performing an overwriting over label widget. Example:
python - Update Tkinter Label from variable - Stack Overflow When you change the text in the Entry widget it automatically changes in the Label. from tkinter import * root = Tk () var = StringVar () var.set ('hello') l = Label (root, textvariable = var) l.pack () t = Entry (root, textvariable = var) t.pack () root.mainloop () # the window is now displayed
Update Tkinter Label from variable - tutorialspoint.com #import the required library from tkinter import * #create an instance of tkinter frame win = tk() win.geometry("750x250") #create a string object and set the default value var = stringvar() #create a text label label = label(win, textvariable = var, font= ('helvetica 20 italic')) label.pack() #create an entry widget to change the variable value …
How to update Tkinter labels using a button. - Python We don't have the file so don't know what it contains, nor did you say how you want the labels updated. Initially you open "stats" as read only and never close it. When you open it again in the write() function, the result is unpredictable because the file is already open.
How to update a Python/tkinter label widget? - tutorialspoint.com Output. Running the above code will display a window that contains a label with an image. The Label image will get updated when we click on the "update" button. Now, click the "Update" button to update the label widget and its object.
How to update the image of a Tkinter Label widget? - tutorialspoint.com In the following example, we will create a button to update the Label image. #Import the required library from tkinter import* from PIL import Image, ImageTk #Create an instance of tkinter frame win= Tk() #Define geometry of the window win.geometry("750x600") win.title("Gallery") #Define a Function to change to Image def change_img():
Post a Comment for "39 how to update a label in tkinter"